In our previous tutorial we learned about what is react now we will learn how to install reactjs and get started with it. I would suggest to learn little bit about react first before you get started. Checkout the following article:
React can be installed in two different ways:
- Using Node and NPM
- Using CDN Url
Install react using Node and NPM
To work with react you must have following things installed on your computer. If you do not have them no worries I will teach you how to do it:
- a web browser
- npm
- nodejs
Let's start installing nodejs and npm first. I would suggest to install nvm which can manage multiple node and npm versions on your local machine so that you can work on different projects where you need different node versions rather then stuck with one node version on your machine.
It will also be beneficial if you want to upgrade your project to different node versions etc... To install nodejs and npm using nvm checkout my following tutorial. Once you have node and npm up and running you can proceed to the next step:
Install NodeJs and NPM using NVM
Install create-react-app cli tool
Now, we will install create-react-app cli tool to install our react project. Open your teminal window and start typing following command:
# switch to lastest node nvm use node # install create-react-app cli tool globally npm install -g create-react-app # check the version to make sure # installation was successful create-react-app --version # create a react project now create-react-app <project-name> # go to project root dir cd <project-name> # start the react app npm run start
Above command will show following output:
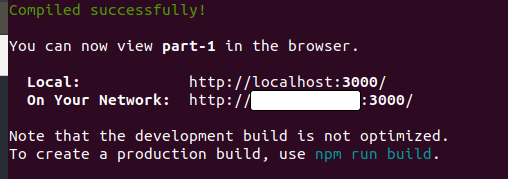
If you copy the url http://localhost:3000 and paste on your browser you will see a react app running.
Install react using cdn url
If you want to install using react cdn urls you can use it like below:
<!DOCTYPE html> <html> <head> <title>Sample React App</title> <!-- Load React. --> <!-- Note: when deploying, replace "development.js" with "production.min.js". --> <script src="https://unpkg.com/react@16/umd/react.development.js" crossorigin></script> <script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js" crossorigin></script> <!-- React with JSX Support --> <script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script> <!--Sample Text--> <script type="text/babel"> ReactDOM.render( <h1>I am React JS!!</h1>, document.body ); </script> </head> <body> </body> </html>
At this point we have react installed and ready to use. In upcoming tutorials I will teach you about react more in detail.