To start building nodeJS app I assume you have all necessary knowledge regarding basics of docker if you do not have it refer to my docker articles:
Create NodeJs app using Dockerfile
To create nodejs app using docker we will follow the steps shown below:
-
We will create a project with three files
- Dockerfile
- index.js
- package.json
Let's open terminal window on your local machine and create package.json file with following contents:
{ "dependencies": { "express": "*" }, "scripts": { "start": "node index.js" } }
Our package.json file contents our project dependencies and a start up command to run our server. Let's now define a index.js file with following contents:
const app = require('express')(); // define home page app.get('/', (req, res) => { res.send('ready to handle connections.'); }); // listen on 8080 app.listen(8080, () => { console.log('Listening on port 8080'); });
In above file we use express framework to define our home page route and then starting our server on port 8080. Next, let's define Dockerfile which will do following tasks for us:
- We will take a base alpine image . i.e. bare minimum linux image
- We will create a working directory
- We will copy our package json file to working dir
- We will install npm packages
- We will run our server using npm command we defined in our package.json file
Open your terminal window and add following contents to our Dockerfile:
# get bare minimum linux image FROM node:alpine # Define working directory WORKDIR /app # Copy package.json file to our app dir in container COPY package.json /app # Install our dependencies RUN npm install # Copy other project files to our container COPY . . # Run a startup command when our container starts CMD ["npm", "start"]
Alright now that we have our Dockerfile ready let's build our custom image. Open your terminal and run following command to build our image:
# change dir to our project root cd docker-example # build a docker image docker build .
Notice the image id at the end of the output:
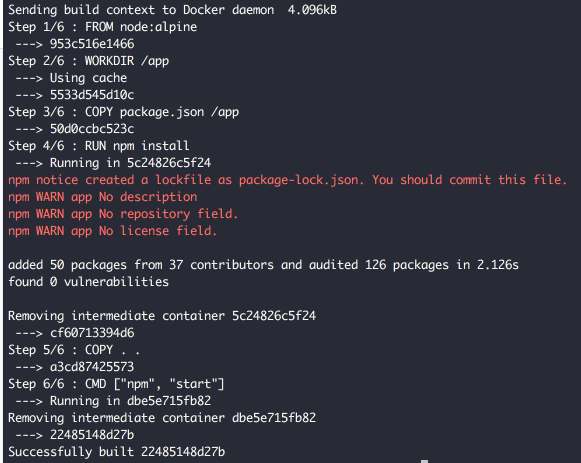
Copy this image id and now let's run our container on host port 9000. Open your terminal and run following command:
# Create and start a container in background # Open 9000 port on host to map to port 8080 on container docker run -p 9000:8080 -d 22485148d27b
Now, open your browser and goto http://localhost:9000 to see your nodejs application running.
Followings are some of the important commands to debug your container:
# check the list of running containers docker ps # login to container bash docker exec -it <container-id> bash # view docker container process logs docker logs -f <container-id> # inspect the container details docker inspect <container-id> # stop the running container docker stop <container-id> # kill the running container docker kill <container-id> # check the stopped/running containers docker ps -a # remove the stopped container docker rm <container-id>
To learn more about how docker process and build the image in more depth read following article:
To get the code for this tutorial checkout following repo on github: