In react it is very crucial to understand how react component works. A component is going through different phases during its life cycle:
- Mounting
- Updating
- Unmounting
Phase diagram can be seen below:
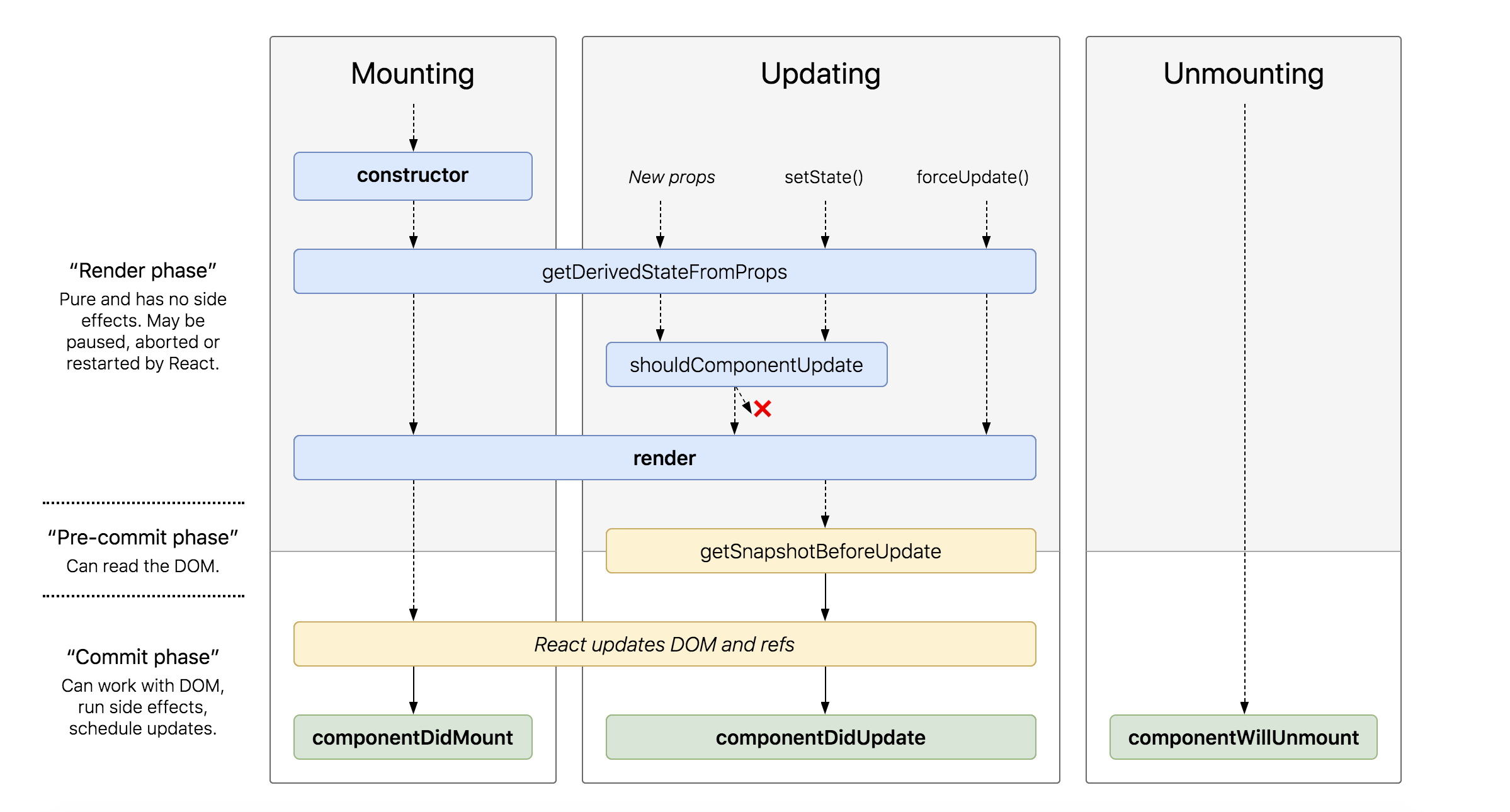
Credit: projects.wojtekmj.pl
Mounting Phase
Mounting meaning putting a rendered component elements into the DOM. When a component is in mounting phase it follows the order below:
- it first calls the constructor() method
- then it calls getDerivedStateFromProps() method
- after that it calls render() method
- once element is mounted using render method it calls componentDidMount() method
The render method is required in a component while other methods are optional if they are defined it will be called in above order.
Let's have a look at the following example:
class HomeComponent extends React.Component {
// STEP-1
// The constructor() method is called with the props
// You should always start by calling the super(props) before anything else
// This will initiate the parent's constructor method and allows the component to inherit methods from its parent
constructor(props) {
super(props);
this.state = { name: null };
}
// STEP-2
// It takes state as an argument, and returns an object with changes to the state
// It is good idea to update state when props changes making sure not to update state
// with same data stored previously compare and then update the state data
static getDerivedStateFromProps(props, state) {
if (props.name !== state.name) {
return {
name: props.name,
};
}
// Return null if the state hasn't changed
return null;
}
// STEP-3
// Method uses props and states and create dynamic html elements
render() {
return <h1>Hello, I am {this.state.name}</h1>;
}
// STEP-4
// When component is mounted on dom this method will be called
// Good place to make api call to populate data on page load
componentDidMount() {
console.log(this.state.name);
}
}
ReactDOM.render(<HomeComponent />, document.getElementById('root'));
Updating Phase
When component is updated with new props or state it goes in updating phase. During update phase component calls methods in following order:
-
getDerivedStateFromProps()
-
shouldComponentUpdate()
-
render()
-
getSnapshotBeforeUpdate()
-
componentDidUpdate()
The render()
method is required all the time and will always be called, the others are optional and will be called if you define them.
Let's have a look at the following example:
class HomeComponent extends React.Component {
// THIS METHOD WONT BE CALLED
// DURING UPDATE PHASE
constructor(props) {
super(props);
this.state = { name: null };
}
// STEP-1
static getDerivedStateFromProps(props, state) {
if (props.name !== state.name) {
return {
name: props.name,
};
}
// Return null if the state hasn't changed
return null;
}
// STEP-2
shouldComponentUpdate() {
// true will call the render
// false will not call the render
return true;
}
// STEP-3
render() {
return <h1>Hello, I am {this.state.name}</h1>;
}
// STEP-4
getSnapshotBeforeUpdate(prevProps, prevState) {
console.log(prevProps, prevState);
}
// STEP-5
componentDidUpdate() {
console.log(this.state);
}
}
ReactDOM.render(<HomeComponent />, document.getElementById('root'));
Un-mounting Phase
When a component is removed from DOM this phase will be activated. During this phase only one method is called:
- componentWillUnmount()
Let's have a look at the following example
class HomeComponent extends React.Component {
// STEP-1
componentWillUnmount() {
console.log("un-mounting from dom");
}
// THIS METHOD WON'T BE CALLED
// DURING UN-MOUNTING PHASE
render() {
return <h1>Hello, I am {this.props.name}</h1>;
}
}
ReactDOM.render(<HomeComponent />, document.getElementById('root'));
I hope by now you have a clear understanding of react lifecycle methods.