When you are working with laravel framework it is important to have some knowledge regarding directory structure of the framework. You should know where to find files you are looking for and where to place classes or third party libraries.
When you download laravel framework for the first time you will see following directory structure.
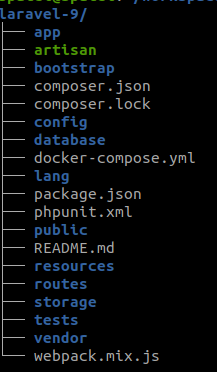
We will learn about each directory and files in depth and why do we need such files.
The App Directory
The app directory is heart of your project where 80% of your code lives. Any files or folder under this directory will be namespaced using App prefix.
Let's look at the directory contents in detail:
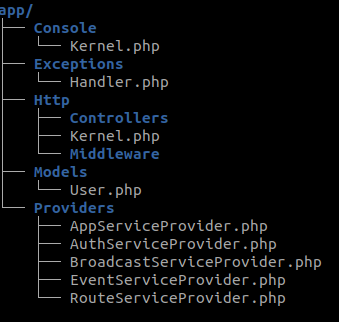
app directory contains additional directories:
-
Console -> where you will write artisan command classes
- Kernel.php -> this is where you schedule background jobs/commands
- Exceptions -> this is where you can write customized exception logic
-
Http -> this directory contains
- Controllers -> laravel controller file lives
- Middlewares -> this is where you will create route middlewares
- Requests -> this is where you can write your request validation classes
- Models -> this is where you will write database models
-
Providers -> providers helps you boot your custom modules or packages
- RouteServiceProvider.php -> loads laravel routes with defined prefix
You can creates more directories inside app directories as per your need in app directory.
The Bootstrap Directory
The bootstrap directory contains following files:
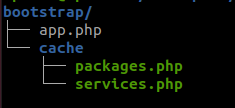
The bootstrap directory contains app.php file which bootstraps the laravel framework. This directory also contains cache directory where framework generated cached files are stored.
When you are running your application in production mode you would have to run some optimization commads like:
- php artisan config:cache
- php artisan event:cache
- php artisan route:cache
All cached file generated because of above commands will be stored under bootstrap/cache folder.
The Config Directory
Laravel uses different types of configuration during runtime. All these configurations are fetched from config folders. It is a good practise to check the files withing this folder and familiarize yourself with different types of configurations as shown below:
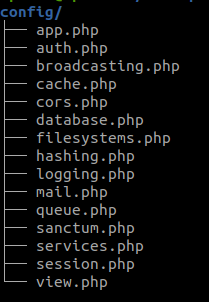
The Database Directory
The database directory contains different directories like:
- factories -> where you can write databse model factories
- migrations -> actual database migration classes
- seeders -> database seeder classes to load initial data
Let's look at the default directory structure:
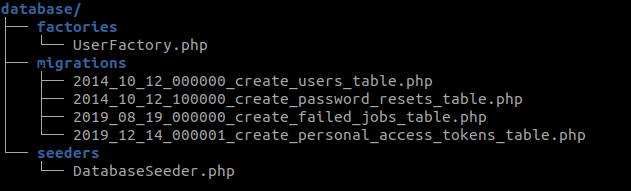
The Lang Directory
The lang directory is the directory where you can find your localization files. You can follow the structure shown below or create more lang translation files in this directory:
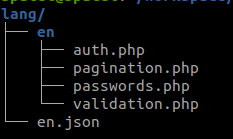
The Public Directory
The public directory contains index.php file which is main entry point for laravel application. This file loads all composer dependencies and then boots the laravel framework.
This is also your web root for your deployed production application. You can store images, css, js or font files in this directory. You can create more files here which are public facing.
One thing to make sure that you do not put any sensitive files in this directory because these files can be accisible from anyone on internet.
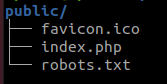
The Resources Directory
The resources directory contains your uncompiled css or js files along with all laravel view files. You can store your application view files in this directory.
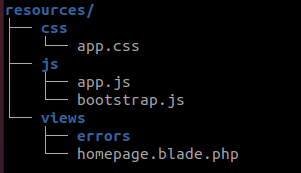
The Routes Directory
This is very important directory in laravel framework. The route directory contains serveral files:
-
web.php
- If your application is not REST API based most likely you will use this file to define your application routes here.
-
all routes defined in this file goes through web middleware by default which means it provides:
- session state
- csrf protection
- cookie encryption
-
api.php
- this file contains all middleware that goes through api middleware by default if you are designing REST api then this is the best place where you can define your REST API routes.
- Routes defined in this file intended to be stateless therefore requests entering in the application should be authenticated based on token and do not have access to sessions
-
console.php
- Generally, laravel allows you to create a console command class and then register this new command via Kernal.php file
- However, it is not necessary to create class file if you are writing less code in your command therefore you can write a closure function instead of creating a class and registering it in Kernal.php file.
- You can create closure function to define your new artisan command rather then writing entire new class in route/console.php file it will be loaded by default by laravel application
-
channels.php
-
The
channels.php
file is where you may register all of the event broadcasting channels supported by your application.
-
The
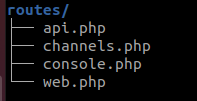
The Storage Directory
The storage directory contains:
- log files
- compiled blade templates
- file based sessions
- cached files
You can also create some other directories in this storage folder which you want to hide from public access on internet. You should create a symbolic link at public/storage
which points to this directory.
You may create the link using the php artisan storage:link
Artisan command.
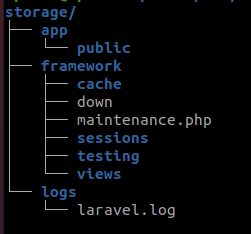
The Tests Directory
In tests directory you can write your feature and unit test for your application. To run tests inside your test directory use following command:
php artisan test
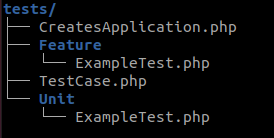
The Vendor Directory
This directory contains all of your third-party libraries installed via composer command.
Other files in root folder
Aside from above directories you will also see some files in laravel application root folder. Following files can be found in your laravel root folder.
- composer.json -> defines your composer dependencies
- composer.lock -> defines locked versions for your third-party libraries defined in composer.json file
- artisan -> bash file that boots your console application and runs artisan commands
- docker-compose.yml -> docker dependencies for your local laravel project
- package.json -> defines your javascript dependencies
- phpunit.xml -> defines your unit test related phpunit configurations
- webpack.mix.js -> defines your commands to mix or minify your js/css files
Hope this tutorial helps you in some way to learn about laravel directory structure.