In our last tutorial we learned about how to create your first page in laravel 9 but now its time to move on and learn laravel in deep.
The first feature that you need to learn in laravel is how to use configuration files and environment variables. We will learn about environment variables later in upcoming tutorial.
In this tutorial I will cover configuration files in depth.
What is config file in laravel? Why do we need to use configurations?
Imagine that you are working on a bigger project where you develop small features or modules. Let say you are suppose to create following modules or features for your blog site.
- comment module
- post module
- user module
- admin module
- tag module
Consider for each module you are going to develop you have to configure some variables. How would you organise your module related variables in laravel?
Laravel has this concept of storing configuration in config directory. It helps you organize your feature/module related configurations.
When you download laravel 9 framework you would see following laravel config files in config folder:
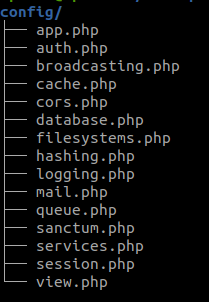
Each file name in this directory refers to some specific module and module related configurations are stored as php array in this file.
Let's take a look at the sample config file for config/service.php file:
<?php return [ /* |-------------------------------------------------------------------------- | Third Party Services |-------------------------------------------------------------------------- | | This file is for storing the credentials for third party services such | as Mailgun, Postmark, AWS and more. This file provides the de facto | location for this type of information, allowing packages to have | a conventional file to locate the various service credentials. | */ 'mailgun' => [ 'domain' => env('MAILGUN_DOMAIN'), 'secret' => env('MAILGUN_SECRET'), 'endpoint' => env('MAILGUN_ENDPOINT', 'api.mailgun.net'), 'scheme' => 'https', ], 'postmark' => [ 'token' => env('POSTMARK_TOKEN'), ], 'ses' => [ 'key' => env('AWS_ACCESS_KEY_ID'), 'secret' => env('AWS_SECRET_ACCESS_KEY'), 'region' => env('AWS_DEFAULT_REGION', 'us-east-1'), ], ];
You can see this file returns array with key/value pair.
For your blog site you can add a new file called blog.php in config folder and add your module configuration in this file as seen below:
<?php return [ /* |-------------------------------------------------------------------------- | Blog Website Moduel Configs |-------------------------------------------------------------------------- | | This is the file where you can store your blog site related configs | */ 'comments' => [ 'enabled' => true, ], 'post' => [ 'enabled' => true ], 'tag' => [ 'enabled' => true ], 'user' => [ 'enabled' => true ], 'admin' => [ 'enabled' => true ], ];
I hope you got my point idea here is to create a config file and then you can add key/value pair as per your need.
Now, we will learn how we can access this config variables in laravel 9.
How to use configurations in laravel?
Let's say that you want to access token from postmark service as seen in above example. Laravel gives you a facade or config function that you can use.
Let's look at the following code to access postmark -> token from services.php file:
# USING LARAVEL CONFIG FUNCTION # get value of services.php -> postmark -> token $token = config('services.postmark.token'); # override configuration on-fly/runtime config(['services.postmark.token' => 'some value]); # USING LARAVEL FACADE Config::get('services.postmark.token'); Config::set('services.postmark.token', 'some value'); # USING LARAVEL MAKE FUNCTION $configService = app()->make('config'); $configService ->get('services.postmark.token'); $configService ->set('services.postmark.token', 'some value');
How to cache configuration in laravel9?
In production/staging environment you might not be changing configuration often. Also to speed up your laravel application you would cache all this configuration in single file.
The way you can cache this configurations in one single file. Use following artisan command:
# to create cache for configuration php artisan config:cache # to break or clear the cached configs php artisan config:clear
If you are beginner and do not know what is artisan command do not worry we will cover each topic in seperate blog article.
For now, just remember that above command will help you speed up your laravel application in production environment.
You can use this locally however during local development you might be adding configuration often and it is not fesible to create cache and then break the cache.
What did we learned today?
By reading above article you have gained some good knowledge about laravel configurations:
- how to create laravel config file? Where to create laravel config file?
- what to store in config file?
- how to access configuration stored in config file?
- how to speed up your laravel app by caching config files?
In our next tutorial we will learn about environment variables.