What is .env file in Laravel 8?
When you are developing a web app you often have different environments to test your web app. For example:
- Local or Development
- Staging
- Production
You may also have different developers working on the same app and they might need to set their environment differently than others. Therefore laravel provides you with .env file that works across different environments.
Note: .env file should not be commited to git project.
Laravel's default .env
file contains some common configuration values that may differ based on whether your application is running locally or on a production web server.
Laravel also provides you .env.example file where you can only put placeholder keys so that different developer or environment can use different values according to their need.
Do not add sensitive information either in .env or .env.example file that would create security issue and some hacker can easily sniff this type of data from your repository.
Consider following diagram:
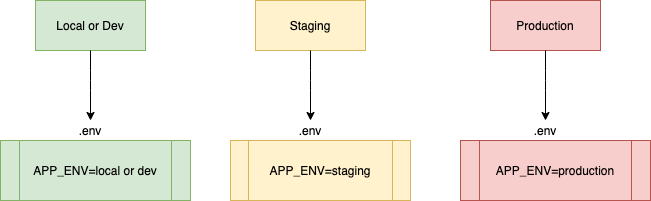
You have three different web environment to test your app. For example: dev/local, staging or production. Each of your environment is using different .env file because we do not commit .env file you have to create this file manually on each environment.
Now, we have set our APP_ENV variable on each environment with different values. Say you want to run certain logic when env is either staging or local you can use following laravel function to check the environment.
if (App::environment('prduction')) {
// The environment is production
// Run your production env specific logic here
}
if (App::environment(['local', 'staging'])) {
// The environment is either local OR staging...
// Run your ocal OR staging env specific logic here
}
How to fetch specific key from .env file in Laravel 8?
Let say you have following keys/values defined in your .env file:
DB_PORT=3306
DB_HOST=mysql
DB_USERNAME=sail
DB_CONNECTION=mysql
DB_PASSWORD=password
DB_DATABASE=example_app
Now, if you want to use one of the above key in your controller or any other classes or file you can use following function to fetch the value:
# print the value of DB_HOST
echo env('DB_HOST', false);
The second value passed to the env
function is the "default value". This value will be returned if no environment variable exists for the given key.
What are configuration files in Laravel 8?
These configuration files allow you to configure things like your database connection information, your mail server information, as well as various other core configuration values.
All of the configuration files for the Laravel framework are stored in the config
directory.
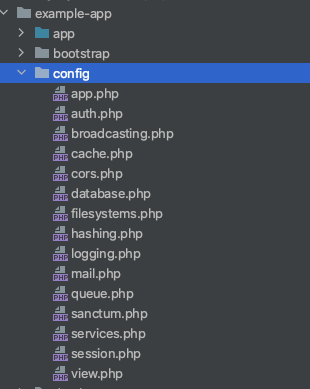
Configuration file uses .env variables to fetch the congiuration dynamically. For example: your database configuration might be different on local, staging or on production server depending on what is stored inside your .env file in each environment.
If you open config/database.php file it might look like following:
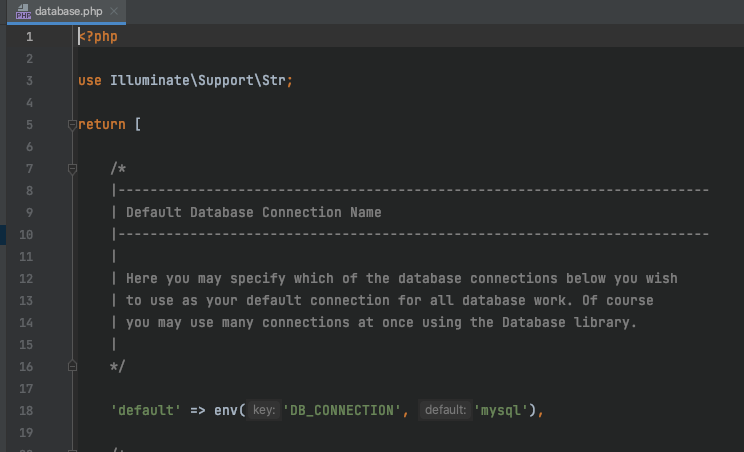
Notice following line:
# if DB_CONNECTION variable is defined in .env
# file than it uses that value otherwise it uses mysql
# as default value
env('DB_CONNECTION', 'mysql');
How to fetch configurations in Laravel 8?
Now, that you know all the configuration files are stored in config folder in laravel 8 project. You are wondering how do I fetch configurations defined in these configuration file.
In laravel configuration file name is used as a key and then whatever variables are defined in that file can be accessed using dot notation. For example:
# fetch database default config $databaseDefaultKeyValue = config('database.default'); # fetch current app timezone $timezone = config('app.timezone', 'Asia/Seoul');
It is also possible that you can also override this values on-fly using following example:
# override what is defined in config/app.php => timezone key
config(['app.timezone' => 'America/Chicago']);
How to cache Laravel 8 configurations?
To boost your laravel app by storing cached configuration you can use following command:
# create cache copy of configurations
php artisan config:cache
How to hide errors in production environment?
The debug
option in your config/app.php
configuration file determines how much information about an error is actually displayed to the user. By default, this option is set to respect the value of the APP_DEBUG
environment variable, which is stored in your .env
file.
According to laravel doc:
For local development, you should set the APP_DEBUG environment variable to true. In your production environment, this value should always be false. If the variable is set to true in production, you risk exposing sensitive configuration values to your application's end users.