Sometime you want to test your application under different scenarios. For example, you want to start with some number of users for x amount of time.
Then you want to increase your load for some x amount of time gradually. This way you can load test your application under different scenarios.
You can see a nice graph as well if you are testing your endpoint in different stages. Let's look at how we can do this using k6:
import{ sleep } from 'k6'; import http from 'k6/http'; export let options = { homePage: { executor: 'ramping-vus', preAllocatedVUs: 10, startVUs: 3, stages: [ { target: 20, duration: '30s' }, // linearly go from 3 VUs to 20 VUs for 30s { target: 100, duration: '0' }, // instantly jump to 100 VUs { target: 100, duration: '10m' }, // continue with 100 VUs for 10 minutes ], }, }, }; export default function() { http.get('https://your-web-app.com'); sleep(1); }
In above example, we have set some option. We are testing our home page we will start with 3 virtual users first. Next, we will linearly go from 3 virutual users to 20 virtual users for next 30s.
Again, we would like to see a big spike therefore we are instantly jump from 20 virtual users to 100 virtual users in no time.
We would then like to see 100 virtual users for next 10 minutes. If you are to draw a chart for above script you will see something like this:
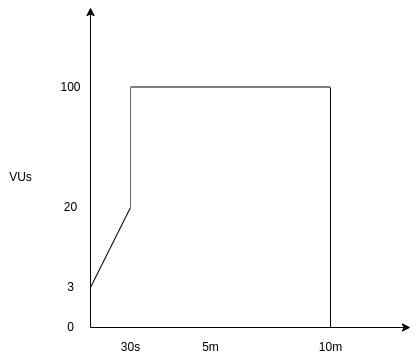
Now, run above script to see the results on your terminal:
k6 run test.js