Sometime, when you are writing a load testing script you want to parse the response html and you want to perform different checks on recieved html.
In this tutorial, we will learn about how to parse response html using k6 and what function we can use to verify some html elements from the response.
Let us assume that you are testing a web page on your localhost website. A response contains following html code.
<article class="active" id="articles"> <ul class="posts"> <li><a href="/a/#" rel="test post">test post</a><date>2 hours</date></li> <li><a href="/a/#" >How to ping and test for a specific port in Linux?</a><date>6 days</date></li> </ul> </article>
Now, we will create a test k6 script that will hit the localhost url and then parse the html. Once we parsed the html we will look for ul with class posts.
We will then find first a tag from this ul element and console.log the innerHTML of the a tag.
import http from "k6/http"; import { parseHTML } from "k6/html"; export default function () { // hit the localhost const response = http.get("http://localhost"); // parse the html body of above response const htmlBody = parseHTML(response.body); // find ul element with posts class const posts = htmlBody.find("ul.posts"); // find the first a tag in above ul element const firstATag = posts.find("a").get(0); // now display the innerHTML of first a tag console.log(firstATag.innerHTML()); }
Now, let us run above test script and see output.
k6 run test.js
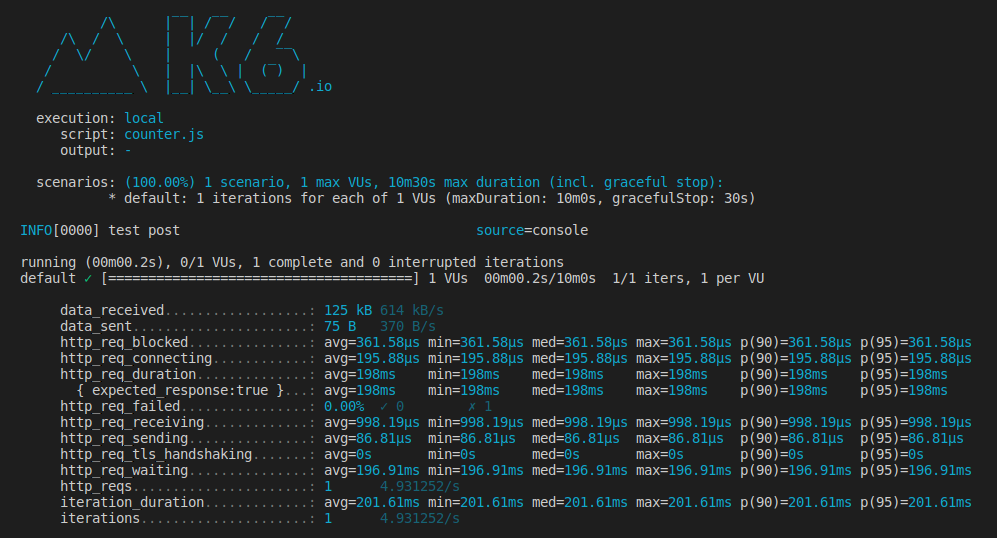
If you look at the above screen you will see INFO[0000] shows test post. You can write meaningful test scripts if you know how to play with html elements.
K6 useful function for html elements
Let say that you have following a tag in your response:
<a href="http://localhost" rel="How to ping and test for a specific port in Linux?">How to ping and test for a specific port in Linux?</a>
You can use following script to check available functions for your a node:
import http from "k6/http"; import { parseHTML } from "k6/html"; export default function () { const response = http.get("http://localhost"); const firstATag = parseHTML(response).find("a").get(0); console.log(firstATag.nodeName()); // a console.log(firstATag.innerHTML()); // How to ping and test for a specific port in Linux? console.log(firstATag.host()); // localhost console.log(firstATag.hostname()); // http console.log(firstATag); }
K6 available functions for html elements
Method | Description |
---|---|
el.nodeName() | Gives you name of the element |
el.nodeType() | Gives you the type of the element |
el.nodeValue() | Gives you the value of the element |
el.id() | Gives you the id of the element |
el.innerHTML() | Gives you html content of the element |
el.textContent() | Gives you plain text content of the element |
el.attributes() | Gives you an array of element attributes |
el.firstChild() | Gives you the first child element of the given element |
el.lastChild() | Gives you the last child element of the given element |
el.children() | Gives you the array of child elements of given element |
el.classList() | Gives you the list of classes as array for the given element |
el.className() | Gives you the class name for the given element |
el.hasAttribute('key') | Check to see if given element has some attribute |
el.getAttribute('key') | Get the given attribute of the given element |
el.querySelector('ul') | Returns the first Element which matches the specified selector string relative to the element |
el.hasChildNodes() | Check to see if given element has some child nodes |