Let say you are testing a script where your response has some json attributes. You want to check if attributes has correct values and then add them to a counter.
Later on you want to create a custom metric with threshold to see if our counter found correct number of attributes.
Let say your endpoint gives you following data:
{ "userId": 1, "id": 1, "title": "delectus aut autem", "completed": false }
Now, let us create a script that checks three elements with above values. If attribute has correct value it adds to the counter.
Counter add function takes boolean value true or false. In our case if attribute has correct value it will add it to counter.
Let's look at following script:
import http from "k6/http"; import { Counter } from "k6/metrics"; // create a new counter with name const attributeCounter = new Counter("Attributes"); export const options = { thresholds: { // use same name as your counter // for counter us count metric Attributes: ["count>=3"], }, }; export default function () { // call your endpoint const response = http.get("https://jsonplaceholder.typicode.com/todos/1"); // check attributes with correct values attributeCounter.add(response.json("id") === 1); attributeCounter.add(response.json("userId") === 1); attributeCounter.add(response.json("completed") === false); attributeCounter.add(response.json("title") === "wrong title"); }
In above script, we are creating a new counter called Attribute. We are then adding a custom metric under thresholds key. This new metric will be called Attribute which contains certain condition.
Once given condition is met it will show checkmark beside this new metric if given condition fails it will show x mark beside this new metric.
In above script three conditions are correct while one is incorrect. Our new metric will show 3 correct counts beside our new metric as shown below.
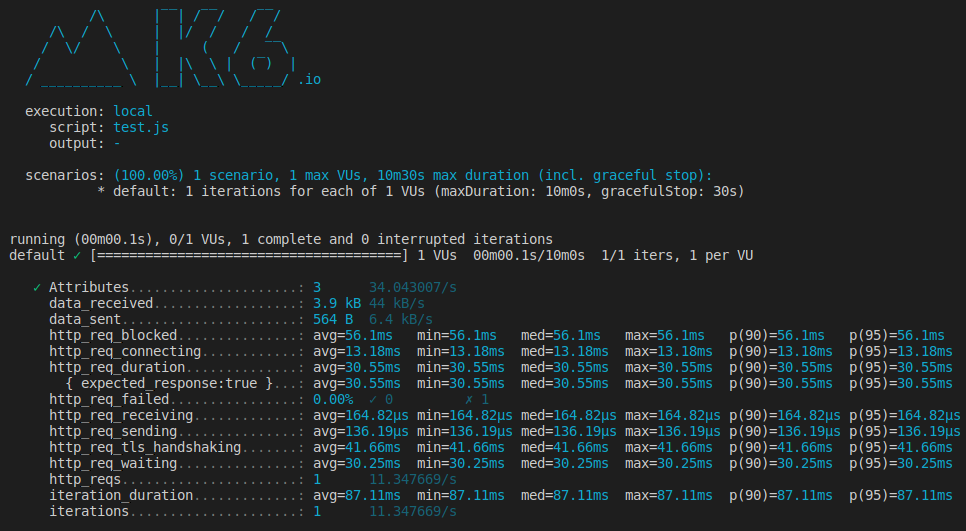
As you can see in above screen shot we have a new custom metric called Attributes which shows checkmark beside it with correct counts.