In our last tutorial we learned basic about Laravel MVC request and response cycle. If you have not followed this tutorial yet I would suggest to go in order I created my tutorials to learn in depth:
In this tutorial we will learn how to create your first laravel static page. As we learned in our last tutorial laravel route is the main entry point in laravel system.
Laravel route point incoming request to specific controller method. When user access specific page in their browser router knows which controller method can handle requested url.
Therefore, let us create our first route. We want to create a homepage for our new website using laravel framework. We have to answer following questions first:
- what would be the route url and name?
- what controller can handle my route request?
- what controller method can handle my route request?
- what would be the name of the view that handles my html code?
Let say that when user hits http://localhost which by default points to /. Slash (/) always refers to root of the website. In our case website root will be our homepage.
We would create a new route in laravel that points to /. We would name our controller HomeController which we have not yet created.
We are just assuming at this point that our HomeController@index method will handle our new route. In this tutorial I assume that you already installed laravel framework locally.
If you have not yet installed laravel framework follow my tutorial on installation:
How to install laravel 9 framework?
How to create a new route in laravel?
All laravel routes are defined in routes/web.php file. Open this file in your favourite editor and let's add following line of code:
<?php use Illuminate\Support\Facades\Route; /* |-------------------------------------------------------------------------- | API Routes |-------------------------------------------------------------------------- | | Here is where you can register API routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | is assigned the "api" middleware group. Enjoy building your API! | */ Route::view('/', 'homepage')->name('homepage');
We have added Route::view method which takes two parameters first is the route and second is the name of the view that handles this request.
At this point we really do not need a controller because our page will be static therefore our route can directly points to view instead of going to controller and then pointing to view.
Later on we will define the controller in this tutorial and we will modify above defined route. Now, if you go to your browser and hit http://localhost you will get following error:
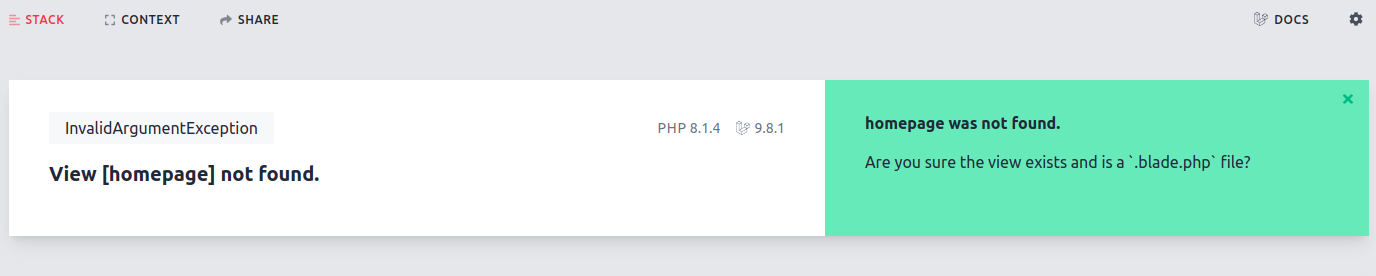
We have got above error because our route now points to a view called homepage however we have not yet created our view file.
Let's create a view file in resources/views/homepage.blade.php with following line of code:
<h1>This is my first laravel page</h1>
Now, we created a new view file. You might be wondering what is this blade.php extention of the file. Well laravel uses blade template engine therefore all view files must have this blade.php extention at the end.
Anyways, now that our view exists we will again hit http://localhost in our browser and this time we will see following output.
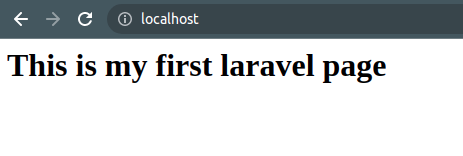
I can see hapiness on your face at this moment because you created your first page. However tutorial is not over yet as I promised now we will learn on creating a controller.
How to define a controller in Laravel 9?
Previously, our route was pointing directly to our view however not in all case you want to do that. A controller can be used for many purpose:
- controller can talk to model and get some data
- controller may intereact with some service that runs business logic
- controller can pass dynamic data to your view file
Therefore, any dynamic pages you want to create should have controller. Let's see how we can create a controller. Go to your project root directory and run following command in your terminal:
# if you are using sail ./vendor/bin/sail artisan make:controller HomeController # if you are not using sail php artisan make:controller HomeController
Above command will create a new controller file in app/Http/Controllers/HomeController.php. Open this file and let us create a new method.
<?php namespace App\Http\Controllers; use Illuminate\Contracts\Foundation\Application; use Illuminate\Contracts\View\Factory; use Illuminate\Contracts\View\View; use Illuminate\Http\Request; class HomeController extends Controller { /** * @description Homepage of website * * @param Request $request * @return Factory|View|Application */ public function index(Request $request): Factory|View|Application { return view('homepage'); } }
Alright, we have added index function in our HomeController class. The first argument is always Request object there is a reason why:
- you can access query params
- you can access post/put params
- you can fetch cookies or user details
- you can access different headers etc..
Request object gives you all necessary data that every web request contains. For now, do not worry about this later on we will learn in depth about Request and Response objects.
Our function calls view method by passing a string homepage in it. View function basically calls the view we have defined earlier.
Alright, now that we have defined our controller class and index method inside. We would change our route to point to this new controller method rather then pointing directly to view file.
Open routes/web.php file and modify our route to point to our new controller method.
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\HomeController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', [HomeController::class, 'index'])->name('homepage');
In above code Route:get function define GET request for route / that points to HomeController@index method. Open your browser and hit http://localhost to see if it is pointing correctly to our new controller.
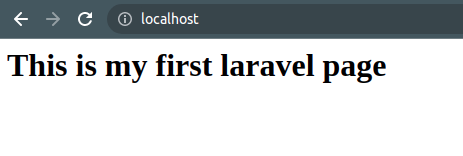
How to pass parameters to laravel view?
Now, you might be wondering how I can pass additional data to my laravel view. Open your controller HomeController class and let's pass a variable called message that we will use in our view file.
<?php namespace App\Http\Controllers; use Illuminate\Contracts\Foundation\Application; use Illuminate\Contracts\View\Factory; use Illuminate\Contracts\View\View; use Illuminate\Http\Request; class HomeController extends Controller { /** * @description Homepage of website * * @param Request $request * @return Factory|View|Application */ public function index(Request $request): Factory|View|Application { return view('homepage', [ 'message' => 'This is my first laravel page' ]); } }
You can see in above code that your view function takes name of the view as first argument and second argument is an array.
You can pass an array of key/value pair to your view file. This passed array keys will be converted to php variable and this variable will hold value that you attached to your key.
In above example in your view you can use $message variable that points to "This is my first laravel page" string. Let's open our homepage.blade.php file and replace harcoded string with following variable.
<h1>{{ $message }}</h1>
Open your browser and hit http://localhost to see if we can see following output.
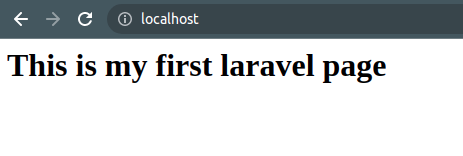
That is it for this tutorial. In next tutorial, we will learn more in depth about laravel routes.
What did we learn today?
Today we have learned following things:
- how to create laravel route
- how to create laravel controller
- what is the purpose of the controller
- how can we create a route that points to either view or controller directly
- what is the template engine laravel use for parsing html file