In this tutorial we will learn something new. Sometime you want to restrict specific users to access your laravel site however in some case you only whitelist ip addresses so that no other ip can access the laravel site.
How to blacklist ip addresses in Laravel?
In first case we want to block only certain users with specific ip addresses. The best place to run this check will be the middleware because when client requests for specific route laravel first goes through route middleware and then hit the controller.
We do not want to hit the controller if we do not want some users to access the site we will show a message to them when they try to reach thee site.
Let's first create a route middleware:
# if you are not using sail
php artisan make:middleware BlockIpAddressMiddleware
# if you are using sail
./vendor/bin/sail artisan make:middleware BlockIpAddressMiddleware
Let's now modified newly created route middleware in app/Http/Middleware/BlockIpAddressMiddleware.php
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class BlockIpAddressMiddleware
{
/**
* @var string[]
*/
public $blacklistIps = [
'192.168.0.5'
];
/**
* Handle an incoming request.
*
* @param Request $request
* @param Closure $next
* @return mixed
*/
public function handle(Request $request, Closure $next)
{
if (in_array($request->getClientIp(), $this->blacklistIps)) {
abort(403, "You are restricted to access the site.");
}
return $next($request);
}
}
Now, that we have our new route middleware let's activate it for all web or api routes.
- all routes/web.php routes go through web middleware
- all routes/api.php routes go through api middleware
So instead of adding middleware to individual routes we would add it globally so that it would get applied to all routes in routes/web.php file.
Open app/Http/Kernel.php:
<?php
namespace App\Http;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel
{
// other code
/**
* The application's route middleware groups.
*
* @var array
*/
protected $middlewareGroups = [
'web' => [
// other middlewares
\App\Http\Middleware\BlockIpAddressMiddleware::class
],
'api' => [
// other middlewares
],
];
}
Let's look at the diagram to understand the clear picture:
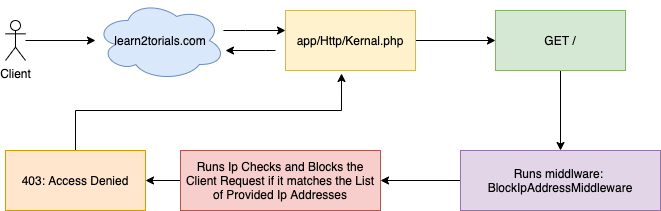
Steps Followed:
- Client with ip: 192.168.0.5 tries to access learn2torials
- Let's assume learn2torials is a laravel website which implements BlockIpAddressMiddleware middlware
- app/Http/Kernel.php finds appropriate route user requests and then run our middlware
- Our middleware checks the ip and respond with 403 error code with appropriate message
- Laravel displays 403 default view or user defined 403 error view
- Client sees 403 error on all the pages he tries to access
Let's consider another scenario:
You are developing some laravel app and you want to allow access to only company users with specific ip addresses and block other users outside your company to access your staging or dev server.
In this case you need to whitelist some ip and block the rest right. Let's create a new middleware for this case:
How to whitelist ip addresses in Laravel?
# if you are not using sail
php artisan make:middleware WhiteListIpAddressessMiddleware
# if you are using sail
./vendor/bin/sail artisan make:middleware WhiteListIpAddressessMiddleware
Let's now modified newly created route middleware in app/Http/Middleware/WhiteListIpAddressessMiddleware.php
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class WhiteListIpAddressessMiddleware
{
/**
* @var string[]
*/
public $whitelistIps = [
'192.168.0.5'
];
/**
* Handle an incoming request.
*
* @param Request $request
* @param Closure $next
* @return mixed
*/
public function handle(Request $request, Closure $next)
{
if (!in_array($request->getClientIp(), $this->whitelistIps)) {
abort(403, "You are restricted to access the site.");
}
return $next($request);
}
}
So instead of adding middleware to individual routes we would add it globally so that it would get applied to all routes in routes/web.php file.
Open app/Http/Kernel.php:
<?php
namespace App\Http;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel
{
// other code
/**
* The application's route middleware groups.
*
* @var array
*/
protected $middlewareGroups = [
'web' => [
// other middlewares
\App\Http\Middleware\WhiteListIpAddressessMiddleware::class
],
'api' => [
// other middlewares
],
];
}
That's all now in this case we toggle the logic so that it only allows ip address from the list of whitelisted ips and block the rest. I hope you are enjoying my tutorials please like comment and share.